This tutorial will guide through the essential steps of enhancing a WordPress site by incorporating custom scripts and stylesheets. The focus will be on how to effectively manage these elements within the site’s structure.
To ensure a clean starting point, it is necessary to first tidy up the functions.php file. This involves removing any code snippets that were previously inserted as part of learning exercises, specifically those illustrating the use of action hooks. Such preliminary code was intended solely for educational purposes to provide a clear understanding of how hooks function within WordPress. The article will proceed with the functions.php file in its simplified form, retaining only the initial PHP tag, setting the stage for the forthcoming instructions on integrating actual, functional code.
Scripts and Stylesheets in WordPress Theme Development
Following the favicon, attention shifts to the stylesheets and scripts of the website, which typically fall into two distinct groups.
The first group comprises the primary stylesheet and script files crafted by the developer. For WordPress sites, custom styles are placed in the style.css file, while the primary JavaScript code is stored in the main.js file.
The second group includes third-party stylesheets and scripts such as:
- normalize.css for standardizing browser default styles;
- bootstrap.css for quick layout, tabs, and accordion implementation;
- superfish.js and superfish.css for crafting accessible drop-down menus;
- modernizr.js for detecting browser capabilities like flexbox and grid.
For this project, a significant number of third-party stylesheets and scripts will be utilized. To organize these resources, a new directory will be created within the ‘nd-dosth’ theme, named ‘assets’. This directory will serve as the repository for all publicly accessible assets, including scripts, stylesheets, images, and custom fonts such as FontAwesome.
Expand the ‘assets’ directory by adding four additional subdirectories to organize the resources effectively. The subdirectories will be named:
Directory | Purpose |
---|---|
css | Stylesheets |
js | JavaScript Files |
images | Visual Assets |
fonts | Typeface Files |
With the directory structure established, it’s time to gather the necessary stylesheets and scripts to advance the project:
- ‘style.css’: This file has already been initialized during the early phase of theme development.
- ‘jquery.js’: WordPress includes the latest version of jQuery by default, eliminating the need for manual download and integration;
- ‘main.js’: This file will be created to hold custom JavaScript code and placed within the ‘assets/js’ directory. The name ‘main.js’ is not obligatory; it could be named ‘custom.js’ or any other preferred term;
- ‘normalize.css’: Acquire the most recent version of this file from Necolas’s GitHub page and place it in the ‘assets/css’ directory;
- Bootstrap files: Obtain the latest Bootstrap package from their official site, unzip it, and transfer the ‘bootstrap.min.css’ to the ‘assets/css’ directory and ‘bootstrap.min.js’ to the ‘assets/js’ directory.
With the files now in the correct locations within the ‘assets’ directory, as seen in the visual code editor, it’s time to implement them.
In WordPress, the best practice for linking scripts and stylesheets to a webpage is through the enqueue system, which is the recommended method for managing these files efficiently.
WordPress Enqueue: Prescribed Way for Styles & Scripts
The WordPress Enqueue System simplifies the process of incorporating scripts and stylesheets into any webpage on a site, using the functions.php file for centralized management. This system eliminates the need to manually insert <link> and <script> tags in each HTML file, a common practice in HTML/CSS projects. Instead, the WordPress Enqueue System automatically generates these tags across all pages based on basic instructions provided by the user.
The term “Enqueue” isn’t exclusive to WordPress; it’s a general English term meaning “to add an item to a queue.” In the context of WordPress, enqueuing refers to the orderly management of stylesheets and scripts, affecting both the frontend and admin aspects of a WordPress site. This concept, as defined by Google, aligns perfectly with its application in WordPress: it’s about organizing and efficiently loading resources as needed.
Managing stylesheets and scripts in WordPress involves the following key steps:
- Sequentially queueing them to control dependencies and create the necessary markup. The sequence of stylesheets and scripts is crucial for a webpage’s functionality.
Regarding stylesheets, it’s common to use a custom stylesheet to modify the styles from third-party CSS frameworks like Bootstrap. Therefore, the Bootstrap CSS should be queued first to allow for these custom overrides.
In terms of scripts, custom scripts often initialize third-party JavaScript libraries such as Bootstrap, Superfish, and Isotope. These third-party scripts should be queued in a specific order, with the custom script placed last. This ensures that all third-party libraries are properly initialized.
Understanding CSS and JavaScript is beneficial for grasping these concepts. However, if you’re not yet familiar with them, don’t worry. These principles will become clearer as you progress through this WordPress Theme Development course.
- The management of scripts and stylesheets in WordPress involves determining the necessity of including specific files on individual webpages throughout the site.
This selective inclusion is essential because not all scripts and stylesheets are required for every webpage to render or function correctly. The WordPress Enqueue System allows for conditional enqueuing, meaning scripts or stylesheets can be specifically targeted to load only on certain pages as needed.
For instance, if a client desires a Masonry layout solely on the homepage and not on other pages, the WordPress Enqueue System can efficiently handle this requirement. The mechanism of how this is accomplished is explained in the subsequent section.
On the frontend, the system primarily relies on the ‘wp_head’ and ‘wp_footer’ action hooks. Internally, WordPress, along with well-crafted plugins, utilize the enqueue system. Theme developers are encouraged to use this system for several reasons:
- Long-term Maintenance: It simplifies ongoing website maintenance;
- Dependency Management: Provides greater control over the order and dependencies of scripts and stylesheets;
- Version Control: Facilitates the management of version numbers, which is crucial for addressing caching issues when making updates, especially on production servers like GoDaddy, Media Temple, etc;
- Centralized Management: New stylesheets or scripts can be added via the functions.php file, avoiding the need to alter multiple files;
- Compatibility: Ensures compatibility and integration with scripts and stylesheets used by plugins.
The next section will delve into the specifics of utilizing the WordPress Enqueue System for efficient theme development.
Key Components of WordPress Enqueue System
The WordPress Enqueue System is a powerful feature that simplifies the integration of scripts and stylesheets. It encompasses two action hooks and two essential functions that facilitate the correct insertion of <script> and <link> tags.
- Front-End Script and Style Management: The wp_enqueue_scripts action hook is pivotal for adding stylesheets and scripts to the front-end pages of a WordPress site. This includes various page types like the Homepage, Feature pages, Blog Archives, and individual Blog Posts;
- Back-End Script and Style Integration: Conversely, the admin_enqueue_scripts action hook is used for incorporating stylesheets and scripts into the back-end pages, such as the Admin Dashboard, individual Page Panels, and Theme Panels;
- Stylesheet Inclusion Function: The wp_enqueue_style() function is essential for registering and enqueuing stylesheets. It not only adds the stylesheets but also correctly generates the corresponding <link> tag. This function ensures that stylesheets are efficiently integrated into your site’s pages, maintaining both functionality and design consistency.
These components collectively form the foundation of the WordPress Enqueue System, offering a streamlined approach to managing website resources. Whether it’s customizing the front-end appearance or enhancing the back-end functionality, these tools provide the flexibility and control needed for effective WordPress theme development.
<link rel="stylesheet" id="normalize-css" href="http://localhost:8888/dosth/wp-content/themes/nd-dosth/assets/css/normalize.css?ver=4.9.9" type="text/css" media="all">
- Script Registration and Integration Function: The wp_enqueue_script() function plays a crucial role in registering and enqueuing scripts within WordPress. It facilitates the automatic generation of the appropriate <script> tags. This function is integral to ensuring that scripts are correctly incorporated into your website, supporting both its functionality and interactive features. By using wp_enqueue_script(), developers can efficiently manage script files, ensuring they are loaded where and when needed across the site.
<script type="text/javascript" src="http://localhost:8888/dosth/wp-content/themes/nd-dosth/assets/js/main.js?ver=1.0.0"></script>
It’s important to differentiate between the role of the wp_enqueue_scripts action hook and the functions wp_enqueue_style() and wp_enqueue_script(). The wp_enqueue_scripts action hook provides the opportunity to enqueue both scripts and stylesheets. However, the actual process of enqueueing is carried out by the wp_enqueue_style() and wp_enqueue_script() functions.
In this guide focused on WordPress Theme Development, our attention will primarily be on using the wp_enqueue_scripts action hook for adding stylesheets to front-end web pages.
We won’t be delving into the admin_enqueue_scripts action hook in this guide. This hook is generally utilized for customizing the WordPress admin interface, and is often a key tool for plugin developers.
Mastering the concept of action hooks is crucial. Once you understand wp_enqueue_scripts, applying the admin_enqueue_scripts action hook for backend customizations becomes straightforward.
Now, let’s dive into how to effectively use the wp_enqueue_scripts action hook in theme development.
Utilizing wp_enqueue_scripts for Frontend Resources
The wp_enqueue_scripts action hook in WordPress is a specialized tool for adding stylesheets and scripts to the front-end of your website. Essentially, it’s one of many action hooks designed to inject data or HTML markup into a front-end webpage.
Its role is singular and clear-cut. During the webpage construction process, WordPress activates this action hook, providing an opportunity to include theme-specific scripts and stylesheets. These are typically inserted into the <head> section of the front-end webpage.
Let’s experiment with it. You can start by inserting the following code into the functions.php file of your WordPress theme.
function nd_dosth_enqueue_styles() {
echo '<link rel="stylesheet" href="http://localhost:8888/dosth/wp-content/themes/nd-dosth/assets/css/normalize.css" type="text/css" media="all">';
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_styles' );
Let’s simplify the explanation: A custom action, nd_dosth_enqueue_styles, has been defined and hooked to the wp_enqueue_scripts action hook. Currently, this custom action is responsible for directly echoing a hard-coded stylesheet <link> tag.
This might raise a question: “Wasn’t WordPress supposed to automatically generate the <link> tag?” Indeed, that is the usual approach. The manual <link> tag will be replaced with the wp_enqueue_style() function shortly, which automates this process.
For the moment, if you go back to the homepage, refresh it, and view the page source, the manually added stylesheet <link> tag should be visible in the <head> element.
Have you ever wondered about the inner workings of the wp_enqueue_scripts action hook in WordPress? How does it seamlessly channel our stylesheet exclusively to the <head> element of the Homepage and not elsewhere, such as the footer?
In the intricate WordPress ecosystem, nothing happens without reason—there’s no magic, only purposeful design.
The wp_enqueue_scripts action hook operates in tandem with the wp_head action hook. Specifically, the wp_head action hook serves as the catalyst for triggering the wp_enqueue_scripts action hook.
Delving deeper, WordPress internally binds its core actions to the ‘wp_head’ action hook. A prime example of this is the wp_enqueue_scripts core action, a connection discernible by inspecting the default-filters.php file within the WordPress core.
An action in WordPress is essentially a specialized PHP function with a distinct objective. In this context, the function of the wp_enqueue_scripts action is specifically to activate the wp_enqueue_scripts action hook. This is analogous to how the wp_head() function initiates the wp_head action hook. To understand this better, you can refer to the implementation of the wp_enqueue_scripts action in the source code.
If the concept seems complex, don’t worry. The key takeaway is this: if you eliminate the wp_head() function from your header.php file, the wp_enqueue_scripts action hook won’t function. Without this action hook, you lose the ability to dynamically add stylesheets or scripts to your WordPress site.
Ultimately, everything links back to the wp_head() function found in the header.php file. This is a crucial aspect of understanding how the wp_enqueue_scripts action hook operates.
Now, with this understanding in place, let’s proceed to replace the traditional stylesheet <link> tag with the wp_enqueue_style() function in WordPress.
Utilizing wp_enqueue_style() to Add and Manage Stylesheets
The fundamental role of the wp_enqueue_style() function is to register and load a stylesheet in the <head> section of a webpage. This function efficiently creates the appropriate stylesheet <link> tag for this purpose.
Internally, wp_enqueue_style() depends on the wp_head action hook to operate effectively.
Furthermore, it accepts five parameters, offering detailed control over how the stylesheet is managed and integrated into the webpage.
wp_enqueue_style( $handle, $source, $dependencies, $version, $media );
Lets delve into the $handle and $source parameters.
The $handle Parameter in Stylesheet Management
The $handle parameter is used to assign a unique identifier or ‘handle’ to your stylesheet, expressed as a string. For instance:
wp_enqueue_style( 'normalize', $source, $dependencies, $version, $media );
You can choose any text that forms a valid PHP string as the handle. Examples like ‘main-style’ or ‘foundation_grid_11’ are completely acceptable in PHP.
However, it’s common practice to name handles in a way that reflects the purpose of the stylesheet. This approach aids future developers or maintainers in understanding the code more easily.
Plugin developers often use the $handle parameter extensively to manage stylesheets, including enqueuing or deregistering them conditionally.
For instance, a stylesheet can be conditionally deregistered using its handle, like:
if( is_page( 74 ) ){
wp_deregister_style( $handle );
}
In the provided example, a stylesheet is deregistered only on a page with the ID 74, using a predefined conditional function called is_page(). In WordPress terminology, is_page() is known as a conditional tag. This term may sound complex, but it simply refers to a function that checks specific conditions.
WordPress offers a variety of conditional tags, and their usage will be covered as the course progresses.
Focusing back on the topic, from a theme developer’s perspective, the $handle parameter is crucial for defining the dependencies of a specific stylesheet. This will be demonstrated shortly.
An example of its practical application includes removing the WooCommerce plugin’s stylesheet for a specialized WooCommerce theme, where the $handle parameter proved to be invaluable.
This scenario, though uncommon, perfectly illustrates the importance and functionality of the $handle parameter.
Specifying Stylesheet Location with the $source Parameter
The $source parameter requires the input of a fully qualified URL where the stylesheet is located. An example of such a URL would be:
wp_enqueue_style(
'normalize',
'http://domain-name.com/wp-content/themes/your-theme/path-to-css-file/normalize.css',
$dependencies,
$version,
$media
);
For theme developers who prioritize adherence to standards and responsible practices: Avoid hard-coding the stylesheet URL in this manner:
http://domain-name.com/wp-content/themes/your-theme/path-to-css-file/normalize.css
Alternatively, a relative path could look like this:
/wp-content/themes/your-theme/path-to-css-file/normalize.css
Both of these approaches may function but lead to increased maintenance complexities over time, making them less advisable.
To streamline development, WordPress offers a convenient function designed to construct fully qualified URLs for various publicly accessible assets such as images, stylesheets, fonts, JavaScript plugins, and more.
This function is:
get_stylesheet_directory_uri()
True to its name, the function simply provides the complete URL of the active theme directory, which, in our scenario, is:
http://localhost:8888/dosth/wp-content/themes/nd-dosth
Observe the absence of a forward-slash ( / ) at the end of the URL. It requires attention when constructing the remaining path to the file.
Now, how do we construct the remainder of the path to the file?
It’s straightforward—we employ PHP concatenation techniques to manually build the rest of the path. For instance:
<?php get_stylesheet_directory_uri() . '/assets/css/normalize.css'; ?>
Observe that the path construction begins with a forward slash.
This highlights a key aspect: traditional PHP techniques and functions can be utilized alongside WordPress-specific functions.
Essentially, WordPress is a large-scale PHP project. Therefore, all the standard practices applicable in custom PHP projects are still valid within the WordPress environment.
Consequently, WordPress interprets the above code as follows:
http://localhost:8888/dosth/wp-content/themes/nd-dosth/assets/css/normalize.css/
It’s important to note the presence of the forward-slash (/) at the start of the path shown in the blue box in the image.
For those new to WordPress development, this detail can be a common oversight.
The function get_stylesheet_directory_uri() returns the complete URL of the active theme’s directory, but importantly, it does not include a trailing forward-slash. Therefore, it becomes the developer’s task to ensure that this forward-slash is correctly added when appending additional parts to the URL.
If consistently adding the forward-slash in conjunction with get_stylesheet_directory_uri() proves challenging, there’s no need to worry.
WordPress offers an alternative function specifically designed to manage these forward-slashes, and this function is:
trailingslashit()
It’s pronounced as “trailing-slash-it.”
To utilize this function, simply supply a URL, and it returns the same URL with a appended forward-slash at the end.
In practical terms within WordPress projects, here’s how we incorporate it.
<?php trailingslashit(get_stylesheet_directory_uri()) . 'assets/css/normalize.css'; ?>
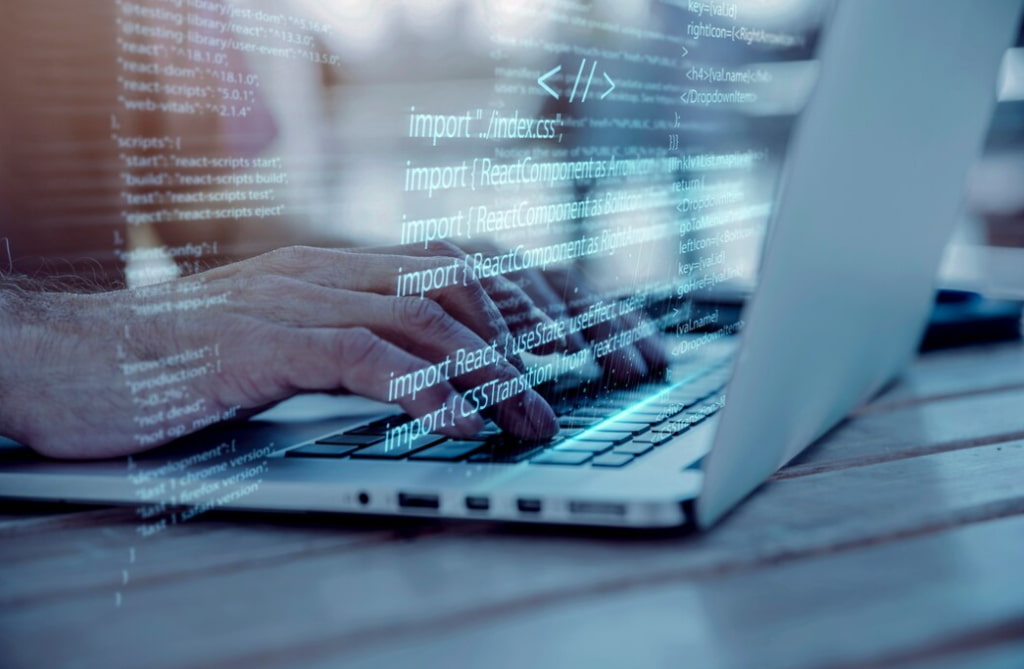
Front-End Integration: Enqueueing for Every Page on Your Website
Let’s move forward to enqueue stylesheets on every front-end page of the website. The explanation for the remaining three parameters of the enqueue function has been intentionally omitted, as they are best understood through practical application.
Shifting focus from theory to practice, the next step involves enqueuing the normalize stylesheet.
To do this, access the functions.php file within the theme. Replace any hard-coded echoes with the following code to enqueue the normalize.css file:
wp_enqueue_style(
'normalize',
get_stylesheet_directory_uri() . '/assets/css/normalize.css',
array(),
false,
'all'
);
In examining the wp_enqueue_style() function call, the first two parameters have already been discussed. Now, let’s delve into the remaining parameters.
The third parameter deals with dependencies. In the case of the normalize.css file, it’s essential to load it before any other stylesheet. However, it doesn’t depend on any other stylesheet, so the $dependencies parameter should be left as an empty array. This explanation might seem a bit abstract at the moment, but the utility of the $dependencies parameter will become clearer when enqueuing the main style.css file.
The fourth parameter relates to the stylesheet’s version number. Including a version number appends it to the stylesheet’s URL as a query string, which is particularly useful for cache busting. This feature becomes important when frequent updates to the stylesheet are anticipated, especially post-launch with caching in effect.
To illustrate, in a practical scenario, a URL incorporating a version number might appear as follows:
http://localhost:8888/dosth/wp-content/themes/nd-dosth/assets/css/normalize.css?ver=1.0
Regarding the normalize.css file, I’ve designated it as a third-party library with no intention of altering its styles. Hence, I set this parameter to false.
Even with this set to false, WordPress generates a version number at the end of the URL, utilizing the current WordPress Software Version (4.9.9 at the time of writing this).
The fifth parameter determines the media or device optimization for the stylesheet. I opt to support all media types, including print media, by choosing ‘all’ for this parameter.
Now, let’s put it to the test. Upon returning to the browser homepage and refreshing, the normalize.css file is indeed seamlessly injected into the <head> element.
The wp_enqueue_style() function is exclusively permitted within the wp_enqueue_scripts, admin_enqueue_scripts, and login_enqueue_scripts action hooks. Attempting to use it outside these action hooks will trigger the following error in WordPress.
Adding the Bootstrap Stylesheet to Your Theme
For incorporating the Bootstrap stylesheet, the process is similar. The Bootstrap enqueue code should be positioned right after the code for enqueuing the Normalize stylesheet.
Below is an example of how the functions.php file would look with the Bootstrap enqueue code included:
function nd_dosth_enqueue_styles() {
wp_enqueue_style(
'normalize',
get_stylesheet_directory_uri() . '/assets/css/normalize.css',
array(),
false,
'all'
);
wp_enqueue_style(
'bootstrap',
get_stylesheet_directory_uri() . '/assets/css/bootstrap.min.css',
array(),
false,
'all'
);
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_styles' );
Upon observation, you’ll notice that, apart from the $source URL and handle parameters, all other parameters mirror those in the enqueue function for the normalize stylesheet.
This similarity arises because, akin to the normalize stylesheet, the bootstrap stylesheet is independent of other stylesheets, and there are no intentions to modify the core styles within the bootstrap.min.css file.
However, these parameters do undergo changes when we enqueue our main stylesheet.
Implementing the Main Stylesheet: Enqueuing the style.css File
To incorporate the main stylesheet, which is the style.css file, insert the following code subsequent to the Bootstrap enqueue function.
wp_enqueue_style(
'main-stylesheet',
get_stylesheet_uri(),
array('normalize','bootstrap'),
'1.0",
'all'
);
We are now fully leveraging the capabilities of the wp_enqueue_style() function. So, without further delay, let’s break it down.
As customary, the first parameter is the handle name.
As for the second parameter, we employ a distinct function to construct the URL for the style.css file. Notably, there is no concatenation of anything at the end of it.
get_stylesheet_uri()
In simple terms, this function yields the fully qualified URL of the style.css file.
“But why this specific function exclusively for the style.css file?” As you’re aware, WordPress anticipates a style.css file in every active theme and mandates placing it at the root level of the theme directory. To facilitate this, WordPress offers the convenient get_stylesheet_uri() function, ensuring the retrieval of the file’s URL. This is one of the reasons I appreciate WordPress!
Moving on to the third parameter, we depart from an empty array. Instead, this time, we furnish two dependencies by specifying the handle names of already registered stylesheets.
array('normalize','bootstrap')
Are the handles listed in the array familiar?
Indeed, these are the handles for the Normalize and Bootstrap stylesheets previously enqueued.
This is a strategic method of instructing WordPress to load the style.css file only after the normalize.css and bootstrap.min.css files have been loaded.
One might wonder why the style.css file depends on Bootstrap and Normalize stylesheets. The answer lies in CSS development practices. Often, it’s necessary to override some Bootstrap framework styles for enhanced control over the website’s layout. The most effective way to do this is by using the style.css file for overrides.
This approach avoids direct modifications to Bootstrap’s core stylesheet, which could lead to complications when upgrading to newer versions of Bootstrap. Considering maintenance, the focus is on long-term time efficiency.
It’s not mandatory to declare the Bootstrap stylesheet as a dependency; this varies with each project. In scenarios where Bootstrap’s layout rules need to be modified, like in the development of a full-width website, declaring it as a dependency is beneficial.
In this course, as part of coding a full-width page later on, the Bootstrap stylesheet is mentioned as a dependency for the main stylesheet in this specific project.
In the fourth parameter, a version number “1.0” is specified.
When the website is moved to a live or production environment, this version number will be updated each time modifications are made to the style.css file. This practice is crucial for cache busting in browsers. By assigning a new version number to a script or stylesheet, the browser is prompted to re-download the file from the server, ensuring that the latest version is used.
Clients often request updates to website styles even after launch, so it’s wise to always include a version number when enqueuing a stylesheet that is likely to undergo changes.
Regarding the fifth parameter, the value “all” is used again. This signals to the browser that the stylesheet is suitable for all types of media/devices.
Here’s how the functions.php file looks with these configurations:
<?php
function nd_dosth_enqueue_styles() {
wp_enqueue_style(
'normalize',
get_stylesheet_directory_uri() . '/assets/css/normalize.css',
array(),
false,
'all'
);
wp_enqueue_style(
'bootstrap',
get_stylesheet_directory_uri() . '/assets/css/bootstrap.min.css',
array(),
false,
'all'
);
wp_enqueue_style(
'main-stylesheet',
get_stylesheet_uri(),
array('normalize', 'bootstrap'),
"1.0",
'all'
);
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_styles' );
Enqueuing the initial stylesheets is now accomplished. As the Dosth project progresses, the functions.php file and the nd_dosth_enqueue_styles() action function will serve as touchpoints for enqueuing more stylesheets based on project needs.
Next, let’s move forward with the enqueuing of our initial scripts.
Mastering Script Enqueue with wp_enqueue_script() in WordPress
The wp_enqueue_script() function is a pivotal tool for registering and enqueueing scripts in WordPress, strategically placing them in both the <head> element and the footer of a webpage. This function effortlessly generates the necessary <script> tag for seamless integration.
Utilizing the syntax: wp_enqueue_script( $handle, $source, $dependencies, $version, $in_footer );, script enqueuing closely parallels the process of enqueuing a stylesheet, with a crucial distinction highlighted in bold in the provided code.
Like its counterpart for stylesheets, this function accepts five parameters. The first four parameters mirror those of the wp_enqueue_style() function. However, the final parameter differs, as wp_enqueue_script() introduces the $in_footer parameter in place of the $media parameter.
Given that the script tag doesn’t support the media attribute, and JavaScript is render-blocking, strategic placement of scripts becomes imperative. Placing scripts just before the closing </body> tag, in the footer, enhances webpage performance by allowing the browser to render the page before executing scripts.
The $in_footer parameter is the key to achieving this placement. By setting it to true, the script tag is placed in the footer; setting it to false results in the script tag being positioned inside the <head> element.
While wp_enqueue_style() relies on the wp_head action hook, wp_enqueue_script() extends its reliance to both wp_head and wp_footer action hooks. The latter is particularly crucial for enqueuing scripts to the footer, ensuring optimal website performance.
With these insights, let’s embark on the script enqueuing journey to enhance our WordPress project.
Exercise: Enqueueing the main.js File
Here’s a brief exercise for you. Enqueuing a script follows a similar process to enqueuing a stylesheet.
During this lesson, we also crafted the main.js file. Now, go ahead and enqueue the main.js file using the following four components:
- wp_enqueue_script() function;
- wp_enqueue_scripts action hook;
- nd_dosth_enqueue_scripts() action.
Enqueue the main.js file to the footer of a webpage using the $in_footer parameter
Take your time to complete this exercise before moving forward.
Once done, you might wonder, “Why do we need a new action like nd_dosth_enqueue_scripts()? Can’t we just enqueue the script using the same nd_dosth_enqueue_styles() action?”
Do you mean something like this:
roject, it’s uncertain who will work on our code next. WordPress, designed with code modularity and readability in mind, allows us to attach any number of actions to the same action hook.
It’s important to note that scripts and stylesheets serve different purposes. This exercise aims to familiarize you with the principles of code modularity and readability, which play a pivotal role in the WordPress development ecosystem.
Here is the updated version of my functions.php file, now incorporating the code for enqueuing the main.js file.
<?php
function nd_dosth_enqueue_styles() {
wp_enqueue_style(
'normalize',
get_stylesheet_directory_uri() . '/assets/css/normalize.css',
array(),
false,
'all'
);
wp_enqueue_style(
'bootstrap',
get_stylesheet_directory_uri() . '/assets/css/bootstrap.min.css',
array(),
false,
'all'
);
wp_enqueue_style(
'main-stylesheet',
get_stylesheet_uri(),
array('normalize', 'bootstrap'),
"1.0",
'all'
);
wp_enqueue_script(
'main-js',
get_stylesheet_directory_uri() . '/assets/js/main.js',
array(),
'1.0.0',
true // load this script in the footer
);
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_styles' );
You’re familiar with each parameter’s role in the code. If the provided code is functioning as expected, we should observe a script tag linking to the main.js file.
Given that we specified true for the fifth parameter, WordPress is expected to enqueue this script in the footer, just before the closing </body> tag.
It’s worth noting that we are currently not enqueuing the bootstrap.min.js file, as its usage in this project is uncertain. However, we’ve prepared it for potential future use.
To verify the code’s effectiveness, let’s test it by visiting the Homepage in the browser and inspecting its page source.
Oh no! Something seems to have gone wrong once again. The main.js script tag isn’t visible in the footer portion of the webpage.
“Oh, come on! What’s the issue now?”
My apologies!
This is occurring because we haven’t informed WordPress about the location of the footer portion on the webpage.
If you recall, in the previous lesson, we faced a similar issue when enqueuing stylesheets. To address it, we specified the location of the header portion by placing the wp_head() function just above the closing </head> tag inside the header.php file.
Likewise, we need to inform WordPress about the location of the footer portion on the webpage.
To resolve this issue, we simply need to insert the wp_footer() function within the footer.php file. Let’s go ahead and implement this fix.
Open the footer.php file and insert the following code.
<?php wp_footer(); ?>
“That’s it?”
Absolutely, that’s it.
Similar to how the wp_head() function triggers the wp_head action hook, the wp_footer() function triggers the wp_footer action hook.
The wp_enqueue_script function relies on both of these action hooks to effectively enqueue scripts to the header and footer sections of the webpage.
Clear, isn’t it? This serves as our way of notifying WordPress, saying, “Hey, WordPress! This spot right here is the footer portion of a webpage. Feel free to enqueue scripts here.”
Now, if we return to the browser and refresh the Homepage’s source once again, this time we should witness the main.js script properly enqueued to the footer section. Oh, and one more thing – let’s not forget to enqueue the jQuery library.
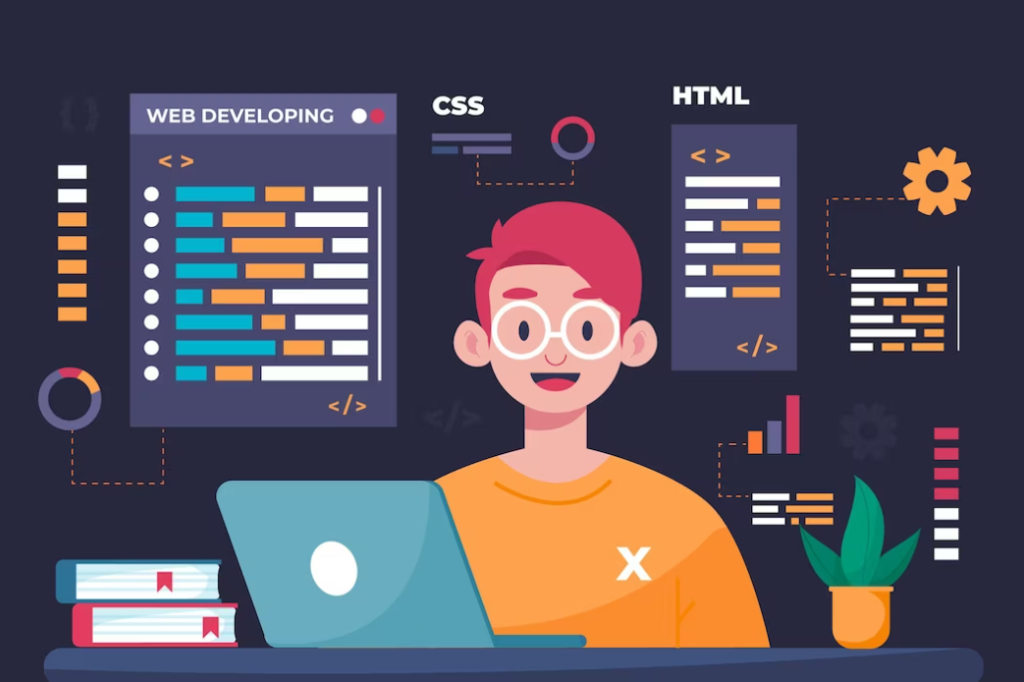
Enqueuing the jQuery Library for Your Theme
The foundation of our theme relies on jQuery, and fortunately, WordPress comes equipped with the latest version of the jQuery library.
WordPress seamlessly enqueues the jQuery script to a frontend webpage whenever another script declares a dependency on it. For instance, by specifying the jQuery script as a dependency for our main.js script, WordPress automatically generates the jQuery script tag just before the main.js script tag in the webpage.
“But wait! I don’t know the handle for the jQuery script. How can I find it?” Excellent question! The handle for jQuery is simply ‘jquery.’ You can confirm this information on the following page of the WordPress Codex: WordPress Codex – wp_enqueue_script
As evident, WordPress comes bundled with numerous other scripts, each accessible for enqueueing using its designated handle.
Now that you’re aware of the handle for jQuery, proceed to specify it in the main.js enqueue call, as follows:
function nd_dosth_enqueue_scripts() {
wp_enqueue_script(
'main-js',
get_stylesheet_directory_uri() . '/assets/js/main.js',
array('jquery'),
'1.0.0',
true
);
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_scripts' );
Upon inspecting the page source of our Homepage in the browser, it’s evident that WordPress is loading the jQuery library within the <head> element.
Surprising, isn’t it? WordPress, along with numerous experienced frontend developers, advocates placing jQuery within the <head> element rather than the footer.
Hence, WordPress enqueues the jQuery script to the <head> element.
“But what if I prefer it in the footer?”
It’s straightforward – download the jQuery script from the jQuery website and enqueue it like any other script.
In certain cases, clients may specifically request loading jQuery in the footer and from a CDN. In such instances, we can enqueue jQuery using the following approach:
wp_deregister_script('jquery');
function nd_dosth_enqueue_scripts() {
wp_enqueue_script(
'jquery',
'https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js',
array(), // no dependencies
null, // you don't need to specify version number
true, // load jquery in the footer
);
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_scripts' );
To prevent any inadvertent enqueuing of the WordPress-provided jQuery, it’s crucial to first deregister it. Following that, you can proceed to enqueue your CDN-based jQuery, treating it like any other script.
Important Note: This technique is specific to all the scripts that WordPress includes by default.
“I have another question!”
Go ahead!
“I just noticed that WordPress also includes the ImagesLoaded JavaScript plugin. I frequently use it, and none of my other scripts rely on it. How can I enqueue this?”
It’s simple – you just need to utilize the wp_enqueue_script() function, providing the handle of the script, like this:
wp_enqueue_script(‘imagesloaded’);
“That’s all? What about the remaining four parameters?”
You can leave the remaining parameters to WordPress – it will manage them for you. No need to concern yourself with those details.
Exactly! WordPress has a knack for knowing where to enqueue. It consistently makes sound decisions on our behalf.
As for the ImagesLoaded JavaScript plugin, I typically don’t use it, so I won’t be enqueuing it. However, if you find it beneficial, feel free to incorporate it into your setup.
Here’s the concluding version of my functions.php file for this lesson:
<?php
function nd_dosth_enqueue_styles() {
wp_enqueue_style(
'normalize',
get_stylesheet_directory_uri() . '/assets/css/normalize.css',
array(),
false,
'all'
);
wp_enqueue_style(
'bootstrap',
get_stylesheet_directory_uri() . '/assets/css/bootstrap.min.css',
array(),
false,
'all'
);
wp_enqueue_style(
'main-stylesheet',
get_stylesheet_uri(),
array('normalize', 'bootstrap'),
"1.0",
'all'
);
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_styles' );
function nd_dosth_enqueue_scripts() {
wp_enqueue_script(
'main-js',
get_stylesheet_directory_uri() . '/assets/js/main.js',
array('jquery'),
'1.0.0',
true
);
}
add_action( 'wp_enqueue_scripts', 'nd_dosth_enqueue_scripts' );
Conclusion
Phew… that concludes this lesson. You’ve done an excellent job. Believe me, if you’ve reached this point in the course, you’ve already grasped 50% of WordPress Theme Development. Now that we’ve tackled the initial stylesheets and scripts crucial for our project, the next lesson will delve into the remaining header elements, kicking off with the <title> tag. You might be interested in an article about key factors for outsourcing WordPress development services.